Export SQL Query Results to Excel: Simplified Guide
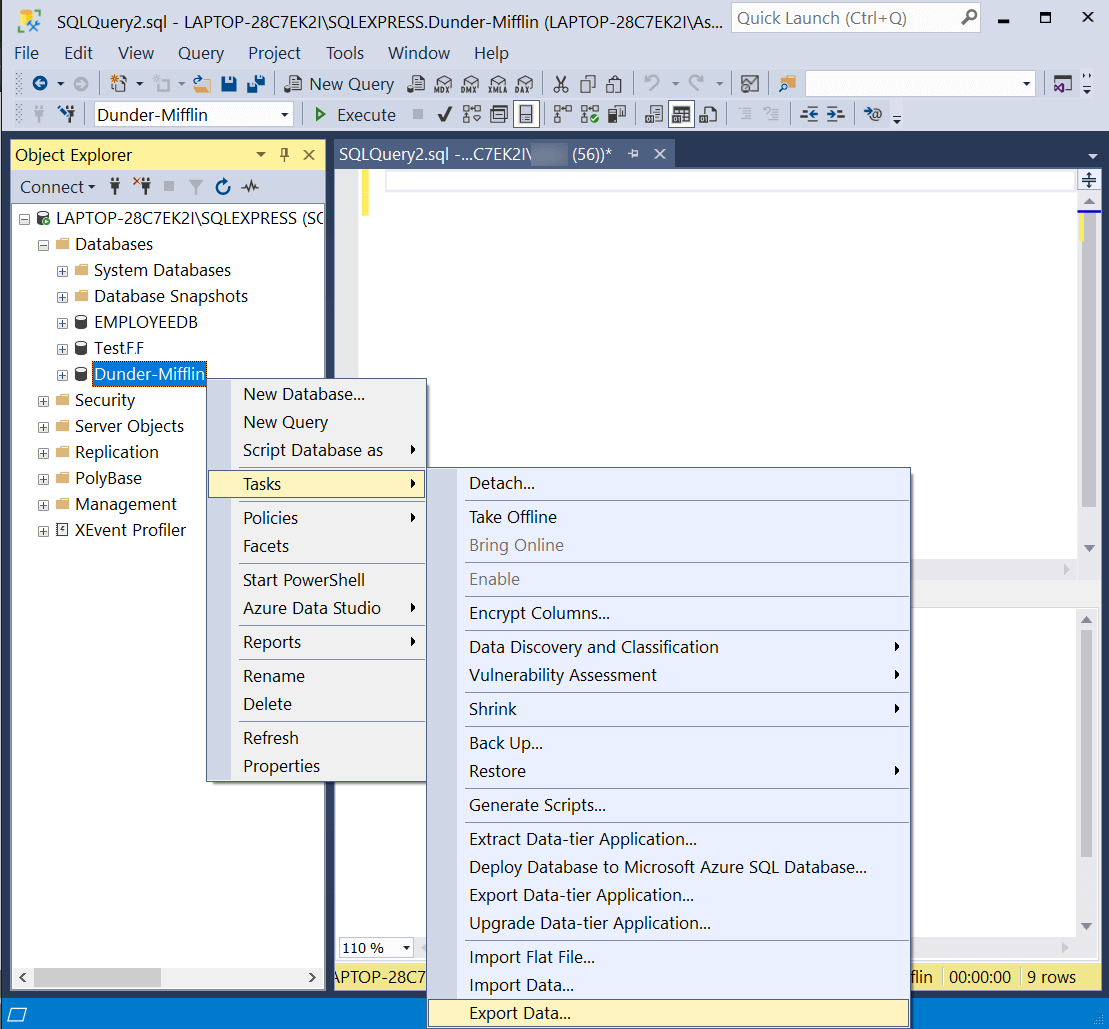
Exporting SQL Query Results to Excel is an essential skill for data analysts, database administrators, and anyone who frequently works with databases. Whether you're pulling data for reporting, analysis, or simply architing data to share with others, converting SQL results into Excel format makes the data more accessible and easier to manipulate. This guide will walk you through different methods to export your SQL queries to Excel, providing you with a toolkit that suits various needs and environments.
Understanding the Export Process
Before diving into the methods, it’s important to understand what happens when you export SQL query results to Excel:
- Database Query: You first run a SQL query on your database to retrieve the data.
- Export: The results of the query are then exported in a format that can be read by Microsoft Excel.
- Format Conversion: Often, data types might need to be adjusted to fit Excel’s structure.
Method 1: Using SQL Server Management Studio (SSMS)
Microsoft SQL Server Management Studio (SSMS) offers built-in functionalities to export query results directly to Excel:
- Open SSMS and connect to your SQL Server.
- Write your SQL query in a new query window.
- After executing the query, right-click on the result grid.
- Select "Save Results As". Choose "Excel" or "CSV" for direct export.
⚠️ Note: Ensure your result set isn't too large; SSMS can be resource-intensive with very large datasets.
Method 2: T-SQL Script for CSV Export
If you need automation or are working with remote access where direct Excel export isn’t feasible, using T-SQL to generate a CSV file can be handy:
DECLARE @Command VARCHAR(8000)
SELECT @Command = 'BCP "SELECT * FROM YourTable" QUERYOUT "C:\YourDirectory\Output.csv" -c -t, -r\ -S YourServer -U YourUsername -P YourPassword'
EXEC master.dbo.xp_cmdshell @Command
A T-SQL script like the above will export your table or query result to a CSV file, which can then be easily imported into Excel:
- Replace `YourTable` with your table name or query.
- Modify the path, server, username, and password accordingly.
Method 3: Using Power Query in Excel
Power Query in Excel is a powerful tool for extracting, transforming, and loading (ETL) data:
- Open Excel.
- Go to the "Data" tab, then click "Get Data" > "From Database" > "From SQL Server Database".
- Enter your server name and optionally the database name, then authenticate.
- Choose your query or select from available tables. Power Query will load the data.
- Once loaded, you can directly work with this data in Excel or refresh it to get the latest updates.
Method 4: Python Script with PyExcel and SQLAlchemy
For users proficient in Python, this method combines the power of SQLAlchemy for database interactions with PyExcel for Excel manipulations:
import sqlalchemy
from sqlalchemy import create_engine
import pyexcel_xlsx
# Create connection
engine = create_engine('mssql+pymssql://username:password@server/database')
# Define your query
query = 'SELECT * FROM YourTable'
# Execute query and fetch results
results = engine.execute(query).fetchall()
# Write results to Excel
pyexcel_xlsx.save_data("your_export.xlsx", {'Sheet1': results})
Ensure you have `sqlalchemy` and `pyexcel-xlsx` installed before running this script:
- Modify the connection string to suit your SQL Server setup.
- Adjust the query as needed.
🚀 Note: This method offers flexibility for more complex data transformations before export if required.
Considerations for Large Data Volumes
When dealing with large datasets, consider:
- Performance: Choose methods that are less resource-intensive or can be optimized for large data transfers.
- Format: CSV might be more efficient than Excel for very large datasets due to less overhead.
- Automation: Automate exports if you need regular updates or real-time data syncing.
In summary, exporting SQL query results to Excel involves several approaches each suited to different scenarios. SSMS is straightforward for on-the-fly exports, T-SQL for automation, Power Query for interactive ETL, and Python for advanced data manipulation before export. Choose your method based on your data volume, complexity, and what fits into your workflow.
What is the fastest method to export SQL results to Excel?
+The fastest method typically involves using direct export options in SSMS or T-SQL scripts if automation is required. CSV exports are generally quicker than Excel files.
Can I automate the SQL to Excel export process?
+Yes, automation is possible through T-SQL scripts with BCP, SQL Agent Jobs, or custom scripts in languages like Python.
How can I handle special characters in my SQL results?
+Excel often struggles with special characters. When exporting to CSV, ensure you specify Unicode encoding to handle these characters. For Excel exports, you might need to pre-format or clean the data before export.
What if my SQL query results contain date or time data?
+Excel can handle dates well, but you might need to format them in SQL or during import to ensure they are recognized as dates rather than strings. Consider using CAST or CONVERT functions in SQL for formatting.
Are there any security concerns when exporting to Excel?
+Yes, ensure that sensitive data is appropriately masked or encrypted. Also, consider access rights when sharing the Excel file and use secure methods for data transfer if it contains personal or sensitive information.