Add a Search Bar in Excel Easily
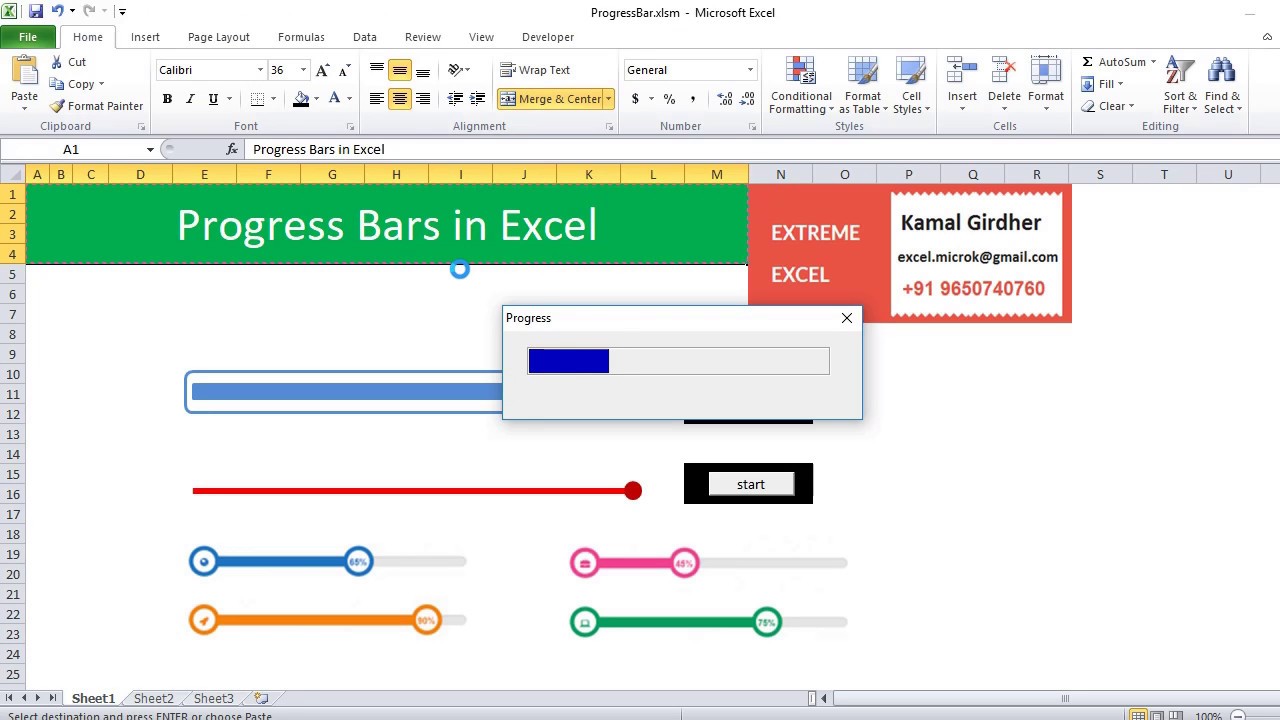
Adding a search bar to your Excel worksheet can significantly enhance its usability, especially when you're dealing with large sets of data. This feature allows users to quickly locate specific data points by filtering through rows or columns based on user input. In this blog post, we'll guide you through the process of adding a search function in Excel using Visual Basic for Applications (VBA).
Prerequisites
- Microsoft Excel (2010 or later versions).
- Basic understanding of Excel functions.
- Familiarity with VBA is helpful, but not necessary.
Setting Up Your Excel Worksheet
Before you begin with VBA, it’s important to prepare your worksheet:
- Open Excel and select the worksheet where you want to add the search functionality.
- Organize Data: Ensure your data is in a tabular format. For example, column headers should be in row 1, and data should be continuous.
- Column Headers: Name your column headers clearly. These headers will be used in the search filter.
Creating the Search Box
Here’s how to add a search box:
- Select Developer Tab: If the Developer tab isn’t visible, go to Excel Options > Customize Ribbon, check “Developer” under Main Tabs, and click OK.
- Insert a Text Box:
- From the Developer tab, click on “Insert.”
- Choose “Text Box (ActiveX Control).” Draw the text box in your preferred location on the worksheet.
- Insert a Command Button:
- Click “Button” from the same “Insert” menu.
- Draw a button next to the text box to run the search.
Programming the Search Function with VBA
Now, let’s program the search feature:
- Open VBA Editor: Press Alt + F11 or click on the “Visual Basic” button from the Developer tab.
- Insert a New Module: Right-click on any of the objects in the Project Explorer, choose Insert > Module.
- Paste the Following VBA Code:
- Assign the Macro:
- Go back to Excel, right-click the command button, select “Assign Macro.”
- Choose the “SearchBox” macro, and click “OK.”
Sub SearchBox()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1") 'Change "Sheet1" to your sheet name
Dim txt As String, rng As Range, cell As Range
txt = ActiveSheet.OLEObjects("TextBox1").Object.Value ' Adjust "TextBox1" to match your text box name
Set rng = ws.UsedRange
For Each cell In rng
If InStr(1, cell.Value, txt, vbTextCompare) > 0 Then
cell.EntireRow.Hidden = False
Else
cell.EntireRow.Hidden = True
End If
Next cell
End Sub
⚠️ Note: Replace "Sheet1" with the name of your active sheet, and "TextBox1" with the name of your text box.
How to Use the Search Bar
Once everything is set up:
- Type your search term into the text box.
- Click the command button to activate the search.
- Excel will filter your data, hiding rows that do not contain the search term.
Troubleshooting and Tips
- If the search doesn’t work, ensure the VBA code is correctly linked to the command button.
- The search is case-insensitive by default. For case sensitivity, change
vbTextCompare
tovbBinaryCompare
in the VBA code. - To make the search more dynamic, consider adding an event handler to automatically trigger the search when typing in the text box.
💡 Note: Regularly backup your Excel file before making extensive VBA changes.
Adding a search bar to your Excel sheet not only makes your data more accessible but also improves your efficiency in handling and analyzing large datasets. This simple addition can be a game-changer for anyone working with data regularly. Remember, Excel's VBA capabilities are vast, offering even more customization for those willing to explore further.
Can I add a search bar to an existing Excel sheet?
+Yes, you can add a search bar to an existing Excel sheet by following the steps outlined in this guide. Ensure your data is properly structured and then proceed with the VBA setup.
What if my data is in multiple sheets?
+The VBA code provided can be modified to loop through multiple sheets. You would need to adjust the ‘Set ws’ part of the code to cycle through each worksheet where you want the search to apply.
Is it possible to make the search case-sensitive?
+Yes, change the vbTextCompare
to vbBinaryCompare
in the VBA code to make the search case-sensitive.